Getting Started with Microcontroller Programming
4.1 Programming Languages for Microcontrollers
Microcontrollers can be programmed using various languages, each with its own advantages and limitations. The most commonly used languages are Assembly and C.
Assembly Language
Assembly language is a low-level programming language that provides direct control over the hardware. It is specific to a particular microcontroller architecture.Pros:
- High efficiency and speed
- Direct hardware manipulation
- Small code size
Cons:
- Complex and difficult to learn
- Error-prone and tedious
- Non-portable across different microcontroller families
Example:
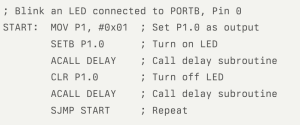
C Language
C is a high-level programming language widely used for microcontroller programming due to its balance between control and ease of use.Pros:
- Easier to learn and write than Assembly
- Portable across different microcontrollers
- Rich set of libraries and functions
Cons:
- Less efficient than Assembly
- Larger code size
- Requires a compiler
Example:
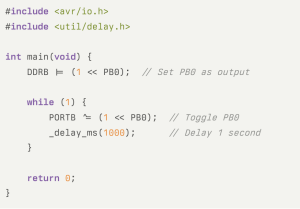
4.2 Development Environments and Tools
Effective microcontroller programming requires a variety of tools to write, compile, debug, and upload code. Here are some of the most popular development environments and tools:
Integrated Development Environments (IDEs)
IDEs provide a unified interface for writing, compiling, and debugging code. Popular IDEs include:
- Arduino IDE: User-friendly, ideal for beginners
- MPLAB X IDE: Used for PIC microcontrollers
- Atmel Studio: Used for AVR microcontrollers
- STM32CubeIDE: Used for STM32 microcontrollers
Compilers and Assemblers
- Compilers: Convert high-level language code (e.g., C) into machine code. Examples include GCC for AVR and XC8 for PIC.
- Assemblers: Convert assembly language code into machine code.
Simulators and Debuggers
- Simulators: Allow testing code on a PC without hardware.
- Debuggers: Help identify and fix errors by providing features like breakpoints and step-by-step execution.
Programmers
- Hardware Programmers: Transfer code from a PC to the microcontroller. Examples include AVRISP for AVR and PICkit for PIC.
- Bootloaders: Software that allows programming via serial or USB without additional hardware.
4.3 Basic Input/Output Operations
Microcontrollers interact with the external world through their I/O ports. Understanding how to perform basic I/O operations is crucial for any microcontroller project.
Digital I/O
Digital I/O pins can be configured as either inputs or outputs.
Example:
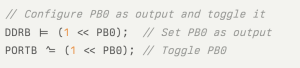
Analog I/O
Many microcontrollers have built-in Analog-to-Digital Converters (ADC) for reading analog signals.
Example:
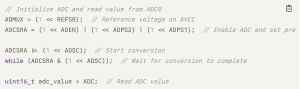
4.4 Simple Programming Examples
Example 1: Blinking an LED
This is the “Hello World” of microcontroller programming. It involves toggling an LED on and off.
Code:
Example 2: Reading a Button Press
This example reads the state of a button and turns on an LED when the button is pressed.
Code:
End-of-Chapter Review Questions
- What are the advantages and disadvantages of using Assembly language for microcontroller programming?
- Describe the role of an Integrated Development Environment (IDE) in microcontroller programming.
- Write a simple C program to toggle an LED connected to a microcontroller pin.
- Explain the difference between digital and analog I/O operations in microcontrollers.
- What tools are essential for debugging microcontroller code?